iOS push notifications
Configure push notifications within Xtremepush and your app
Push notifications for iOS require certificates generated from your Apple Developer account. Follow these steps to enable push notifications:
Get Apple certificates
- Log in to your Apple Developer account at (https://developer.apple.com/membercenter/)
- Select Certificates, IDs and Profiles
- Select Identifiers
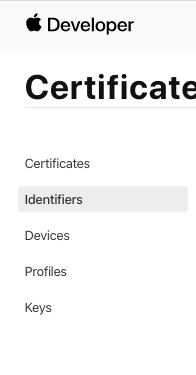
- Select the app you are integrating with Xtremepush and click Edit.
- In iOS App ID Settings, click Edit next to Push Notifications.
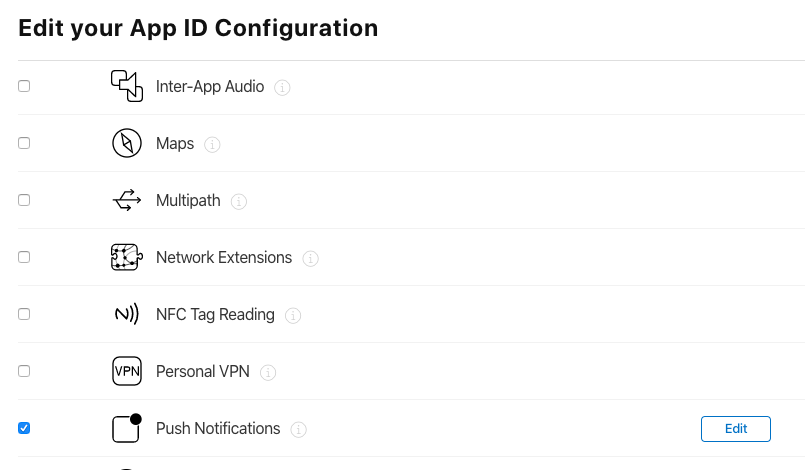
- Under Development SSL Certificate click "Create Certificate" and follow the step-by-step instructions to generate and download the certificate.
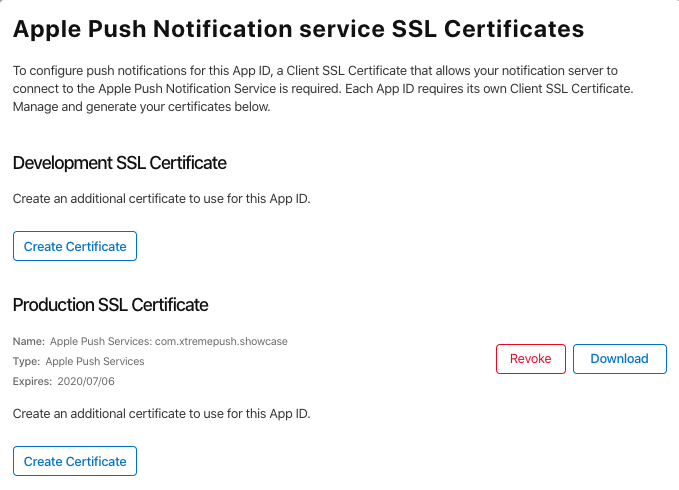
- Repeat this procedure to generate and download a certificate under "Production SSL Certificate".
- Open the certificates, which will show them in the Keychain Access application.
- Select the certification and expand it to see the key below it.
- Hold ⌘Cmd to select both the certificate and key and export them using File > Export Items..., choosing the (.p12) format.
Configure Xtremepush
- In Xtremepush, navigate to **Settings > Apps & sites > click on the matching iOS app > Push > Push Settings.
- Upload the Production and Sandbox (development) certificates and press Save.
Add to app
For basic testing, you can add the SDK's push notification register
function to your didFinishLaunchingWithOptions
method in your Application Delegate. For better user experience, the registration for push notifications should only be triggered after a prompt or value-exchange in your app, so your users know why push permissions are being requested and are more likely to agree to opt in. Learn more in our dedicated guide here.
Xtremepush does not require you to set Delegate of UNUserNotificationCenter as it will conflict with our library.
If you test using development builds then you should turn on Sandbox Mode as Apple uses a different gateway for builds compiled with a development mobile provisioning profile. If you only do enterprise builds you won't need this. Set sandbox mode in a statement that will only run for development builds as it should not be turned on in production.
Inside didFinishLaunchingWithOptions
add the following:
func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplicationLaunchOptionsKey: Any]?) -> Bool {
XPush.setAppKey("XPUSH_APP_KEY")
// Add registration for push notifications
XPush.register(forRemoteNotificationTypes: [.alert, .badge, .sound]);
// Set push notification sandbox mode only for development builds
#if DEBUG
XPush.setSandboxModeEnabled(true)
#endif
XPush.applicationDidFinishLaunching(options: launchOptions)
return true
}
- (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions
{
[XPush setAppKey: @"XPUSH_APP_KEY"];
// Add registration for push notifications
[XPush registerForRemoteNotificationTypes:XPNotificationType_Alert |
XPNotificationType_Sound | XPNotificationType_Badge];
// Set push notification sandbox mode only for development builds
#if DEBUG
[XPush setSandboxModeEnabled:YES];
#endif
[XPush applicationDidFinishLaunchingWithOptions:launchOptions];
}
Add the the following iOS remote notifications handling methods in Application delegate, and place the corresponding Xtremepush method call in each of these as shown.
func application(_ application: UIApplication,
didRegisterForRemoteNotificationsWithDeviceToken deviceToken: Data){
XPush.applicationDidRegisterForRemoteNotifications(withDeviceToken: deviceToken as Data!)
}
func application(_ application: UIApplication,
didFailToRegisterForRemoteNotificationsWithError error: NSError) {
XPush.applicationDidFailToRegisterForRemoteNotificationsWithError(error)
}
- (void)application:(UIApplication *)application didRegisterForRemoteNotificationsWithDeviceToken:(NSData *)deviceToken {
[XPush applicationDidRegisterForRemoteNotificationsWithDeviceToken:deviceToken];
}
- (void)application:(UIApplication *)application didFailToRegisterForRemoteNotificationsWithError:(NSError *)error {
[XPush applicationDidFailToRegisterForRemoteNotificationsWithError:error];
}
Updated almost 3 years ago