Fire real-time events in Android
In-app messages are triggered based on real-time in-app events. Real-time events can also be used for triggering comms like push notifications, email, SMS and inbox messages. This guide describes how to fire events from your Android app.
Custom Events
If you want to send a message to your customers after custom events occur then you must fire these events with the hitEvent(String title)
method. For example, if you want to track as an event whenever a user opens the settings page, you would do it as follows:
mPushConnector.hitEvent("opened_settings");
The event opened_settings
will then be available as a trigger option when creating an Android push message.
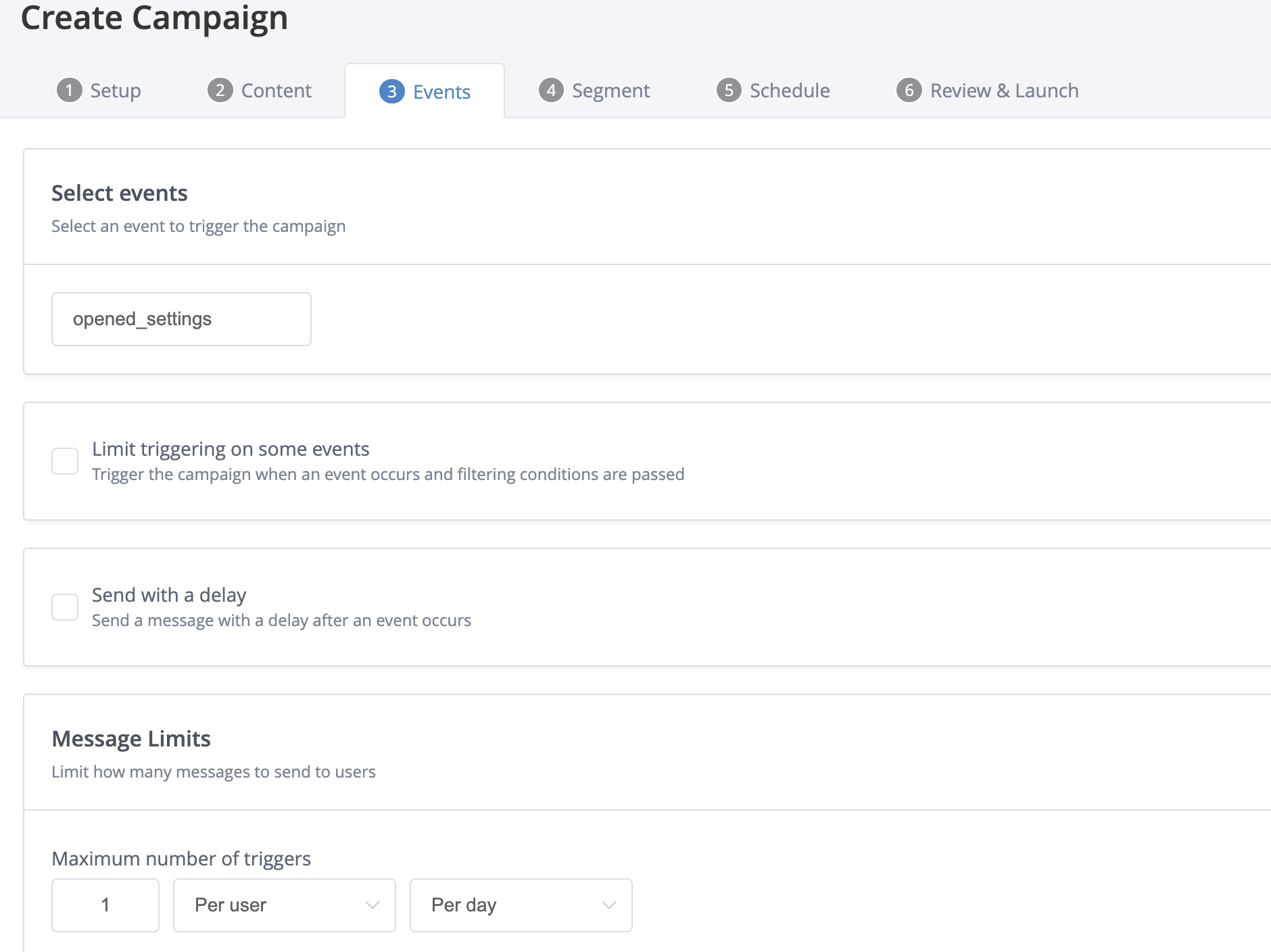
Example of an Android push campaign that will be triggered by the event 'opened_settings'.
An event is created in your project whenever you fire it for the first time from your app (as seen before), or you can also manually create it by navigating to Data Manager > Events > Create event.
Event values
It's possible to pass a value with the event hit. It could be used for additional segmentation and personalisation of your messages.
To pass a string value with the event use:
mPushConnector.hitEvent("test", "payload");
To pass key-value pairs with the event using HashMap:
HashMap <String, String> hm = new HashMap<>();
hm.put("product_category", "Sports");
hm.put("product_name", "Home Jersey");
mPushConnector.hitEvent("product_add_to_basket", hm);
To pass key-value pairs with the event using JSONObject:
JSONObject jo = new JSONObject();
jo.put("type","transfer");
jo.put("amount", 100);
mPushConnector.hitEvent("deposit",jo.toString());
Session Start
There is a default event for tracking session starts or app open events. To do so, call the setEnableStartSession
method before initialising Xtremepush in your main activity:
// INITIALISE THE XTREMEPUSH CONNECTOR HERE
mPushConnector = new PushConnector.Builder("XTREME_PUSH_APP_KEY", "GOOGLE_PROJECT_NUMBER")
.setEnableStartSession(true)
.create(this);
From the Events tab you can then select the trigger Session Start to launch an in-app message.
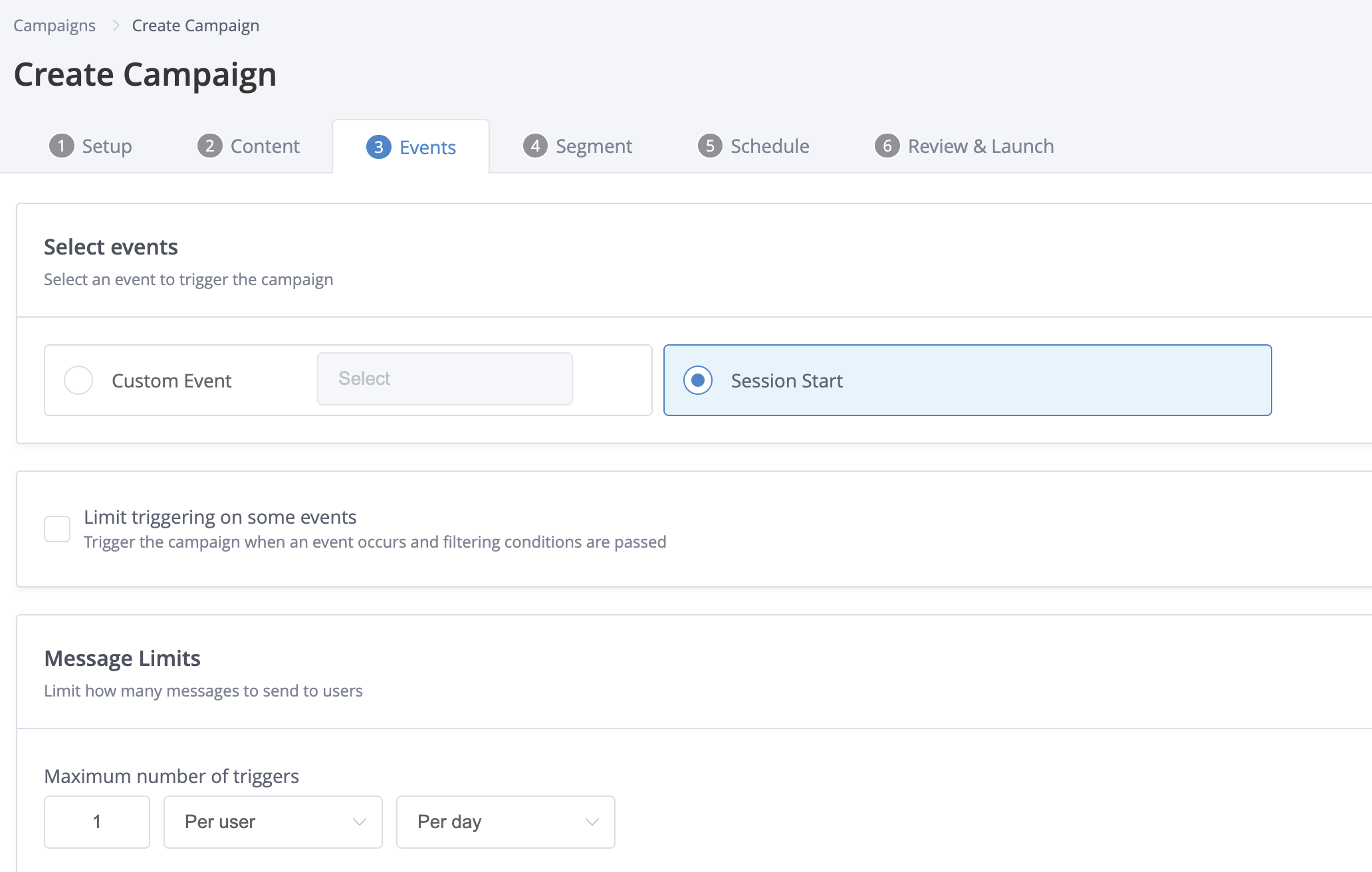
Example of an in-app campaign triggered on Session Start.
Only in-app messages can be triggered on Session Start
Updated 10 months ago