Xamarin.Forms
Integration of Xamarin.Forms requires the steps on this page in addition to those covered in the main Xamarin integration guide.
Integration
There are three files needed to add the Xtremepush functionality to your Xamarin.Forms app. These are the Xtremepush interface, and its implementation for the Android and iOS platforms.
Add the IXPushDelegate to the shared code section of your app.
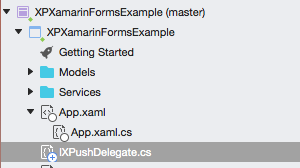
Open the IXPushDelegate
and be sure to change the namespace to your own.
Add the XPushDelegate.cs
files from the Android and iOS folders to the relevant platform section. Again, afterwards, you will need to open these files and change the namespace to your own.
For Android, you will also need to add the nuget package Plugin.CurrentActivity
and initialise the same in your Application class.
Using Xtremepush
Once the project has been set up with a common interface and implementations for each platform, use DependencyService to get the correct implementation at runtime.
Example to call hitTag
:
DependencyService.Get<IXPushDelegate>().HitTag("SampleTag");
The APIs available are:
//sends a single string tag to the platform
DependencyService.Get<IXPushDelegate>().HitTag("TagTitle");
//sends a tag key-value pair tag consisting of title and value to the platform
DependencyService.Get<IXPushDelegate>().HitTag("TagTitle", "TagValue");
//sends an event to the platform
DependencyService.Get<IXPushDelegate>().HitEvent("SampleEvent");
//sends an event key-value pair tag consisting of title and value to the platform
DependencyService.Get<IXPushDelegate>().HitEvent("SampleEvent", "WithValue");
//opens up the Xtremepush inbox
DependencyService.Get<IXPushDelegate>().OpenInbox();
//Sets subscribed to push messages as true or false
DependencyService.Get<IXPushDelegate>().SetSubscription(true);
//Sets the Id associated with the user
DependencyService.Get<IXPushDelegate>().SetUser("UserId");
//Returns an iDictionary containing details about device
DependencyService.Get<IXPushDelegate>().GetDeviceInfo();
//Sends request to XPush to get list of push messages,
//uint offset = amount from latest to return messages
//uint limit = number of messages to be returned
DependencyService.Get<IXPushDelegate>().GetPushList(offset, limit);
//Marks a message as opened (This is tracked automatically so this is not required in most situations)
//string messageId = the id of the message to mark as opened
//DependencyService.Get<IXPushDelegate>().MarkPushAsRead(messageId);
Using GetPushList
Android
On Android, to handle the returned Message list, you must implement the IPushListListener
interface.
This interface has a single method PushListReceived
. An example is shown below.
public class MainApplication : Application, Application.IActivityLifecycleCallbacks, IPushListListener
{
...
public void PushListReceived(IList<Message> p0, Java.Lang.Ref.WeakReference p1)
{
try
{
foreach (Message m in p0){
Log.Debug("XPushExample", "create_time: " + m.Metadata["create_time"]);
}
} catch (Exception e)
{
Log.Debug("XPushExample", "Empty message list received");
}
}
}
iOS
On iOS, in the XPushDelagate.cs file
, GetPushList
takes a callback method as its third parameter. This is set as default, to a method called PushListCallback
, which is also in the file. However you can change this to another method if you wish.
An example of an implementation on iOS:
public void GetPushList(uint offset, uint limit)
{
//replace PushListCallback with your callback function if named differently
XP.XPush.GetPushNotificationsOffset(offset, limit, PushListCallback);
}
public void PushListCallback(Foundation.NSArray arg1, Foundation.NSError arg2) {
try
{
for (nuint i = 0; i < arg1.Count; i++){
XP.XPMessage xpm = arg1.GetItem<XP.XPMessage>(i);
System.Diagnostics.Debug.WriteLine("XPushExample - create_time: " + xpm.Metadata["create_time"]);
}
} catch (Exception e)
{
System.Diagnostics.Debug.WriteLine("XPushExample - Empty message list received");
}
}
Xtremepush Message Object
The XPush
Message object contains the following keys:
- ID: The ID of the message
- Title: The title from the message
- Text: The text of the message
- Campaign ID: The ID of the Xtremepush campaign
- Data: Data includes additional information such as payloads associated with message
- MetaData: Additional data about the message, such as
create_time
andread
(user clicked message)
Deeplinks in Xamarin Forms
The process for deeplinking in the Xamarin Forms application is the same as the standard process to add deeplinks, which is described in our dedicated guide, with a couple of additional changes.
In the Forms project, implement the listener in the format below:
[Application]
public class MainApplication : Application, IInboxBadgeUpdateListener, IDeeplinkListener
{
public MainApplication(IntPtr javaReference, JniHandleOwnership transfer) : base(javaReference, transfer)
{
}
public void InboxBadgeUpdated(int i, Java.Lang.Ref.WeakReference activityHolder)
{
//print out inbox badge
Log.Debug("Inbox Badge is : ", i + "");
}
public override void OnCreate()
{
base.OnCreate();
// If using Xamarin.Forms please install the Plugin.CurrentActivity package
// from NuGet and uncomment the line below
// CrossCurrentActivity.Current.Init(this);
String XPUSH_APP_KEY = "";
String GOOGLE_PROJECT_NUMBER = "";
new PushConnector.Builder(XPUSH_APP_KEY, GOOGLE_PROJECT_NUMBER)
.TurnOnDebugLogs(true)
.SetDeeplinkListener(this)
.Create(this);
}
}
Call the IDeeplinkListener
within the class itself and set the DeepLinkListener within the PushConnector builder:
.setDeeplinkListener(this)
For iOS, the deeplink method needs to be registered as follows:
(void)registerDeeplinkHandler: (XPDeeplinkCallback)callback;
Once those methods are implemented within the Xamarin Forms project, the rest of the deeplink configuration can be taken from the deeplink user guide.
Updated about 2 years ago