React Native
Use the Xtremepush SDK to integrate your React Native apps with the Platform
With the Xtremepush module for React Native, you can add Xtremepush to both iOS and Android apps quickly.
Make sure your mobile apps are added to Xtremepush, following the guides for Android and iOS.
Add the Xtremepush React Native files
Download the Xtremepush React Native files from the React Native SDK releases page, then add the xtremepush.js
file to the root of your project.
Android
Add these files to your Android package:
RNXtremepushReactModule.java
RNXtremepushReactPackage
You will need to add your package name to the top of these files:
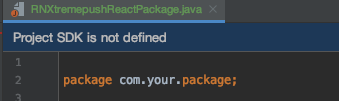
Additionally in your MainApplication.java
function, you should add packages.add(new RNXtremepushReactPackage())
to the getPackages()
function, as shown in this example:
@Override
protected List<ReactPackage> getPackages() {
@SuppressWarnings("UnnecessaryLocalVariable")
List<ReactPackage> packages = new PackageList(this).getPackages();
// Packages that cannot be autolinked yet can be added manually here, for example:
// packages.add(new MyReactNativePackage());
packages.add(new RNXtremepushReactPackage());
return packages;
}
Add the Xtremepush SDK and dependencies by following the guide to Import the Android SDK and then the steps in Integrate the Android SDK.
If you are also enabling push notifications, follow the steps to configure the Firebase server key following the instructions in the Android push notifications guide.
iOS
Add these files to your Xcode project:
RNXtremepushReact.h
RNXtremepushReact.m
Add the Xtremepush SDK and dependencies by following the guide to Import the iOS SDK and then the steps in Integrate the iOS SDK.
If you are also enabling push notifications, you will need to set up your Apple push certificates and add the notifications handling methods described in the iOS push notifications guide.
Using the Native Module from JavaScript
To call the Xtremepush SDK functions from JavaScript using the native module, the following import is required at the top of your JavaScript file:
import {NativeModules} from 'react-native';
Once this has been included, the Xtremepush functions can be called as follows:
NativeModules.Xtremepush.hitTag("tagTitle");
NativeModules.Xtremepush.hitTagWithValue("tagTitle","tagValue");
NativeModules.Xtremepush.hitEvent("eventTitle");
NativeModules.Xtremepush.openInbox();
NativeModules.Xtremepush.setUser("userId");
Alternatively, to avoid typing NativeModules.Xtremepush
each time, this can be simplified by setting a constant once, so that a shorter reference can be used elsewhere in your JavaScript file, for example:
const XPush = NativeModules.Xtremepush;
XPush.hitTag("tagTitle");
Make sure you set the user ID
It is recommended that at this stage you use our SDK method to set user IDs by following our dedicated guide to ensure devices can be associated and targeted in your campaigns by your own unique identifier.
Updated over 3 years ago