Consent Management
Allow users to subscribe to different categories
Subscription Preferences is now Consent Manager
As of May 2024 (release 2024.3.0) the Subscription Preferences page becomes Consent Manager, with some useful updates. This page describes everything you need to know about the immediate changes to the Subscription Preferences feature so you can be ready for the update.
The Consent Manager feature allows you to create different subscription preferences to which your users can subscribe, for example, if you have different newsletters for different topics (such as Business, Lifestyle, News), you can create these topics in Xtremepush and then use them to segment your recipients.
Enabling Consent Management
Consent Management is an optional feature and not enabled by default. If you wish to use this feature please contact us. It is enabled on a project basis.
Available Channels
It's possible to create preferences and set them against Xtremepush channels; these are the channels that require a subscription: SMS, email and push (mobile and web).
Custom channels
With the new Consent Manager, it's also possible to create custom channels which your organisation may use, even if they are not channels offered by Xtremepush. This allows you to keep your central source of truth for all things permissions based inside your Xtremepush project and just reference those preferences wherever else they may need to be checked.
The Channels tab will show the available Xtremepush channels available to the current project, as well as a section where you can add your own custom channels. Once added they will be available to set up new preferences against them in the next tab.
Preferences without channels
It is also possible to set up a preference to track consent for items that do not necessarily link to a channel at all. For example, you might want to ask your users if they consent to you using their data in your machine-learning algorithms in order to offer products tailored to them. To do this, set up a new preference and leave all channels unchecked.
Create the preferences
Once the feature has been enabled on your project, navigate to Data > Consent Manager > Subscription Preferences and click on Create preference to create a new preference. The available fields are:
- Name: The name should avoid spaces and dots, and ideally limit to lowercase letters, digits and underscore. This will allow easier use of the subscription preference through the mobile and web SDKs and the API. Example: football_news.
- Display Name: Use this field to provide a friendly name for the category in the Xtremepush interface.
- Channel: The messaging channels to which the category will be applied.
- Type: This is a required field and you can select two options:
- Marketing: This consent type is used for consent where explicit consent is required before contact can be with the customer, for example, consent to receive sports news.
- Legitimate Interest: This consent type is used for consent where explicit consent is not required before contact can be made with the customer, but the customer has the option to opt-out of these communications, for example, weekly gaming updates.
Single preference across multiple channels
As an improvement from the old Subscription Preferences, the new Consent Manager allows you to cover multiple channels with a single preference.
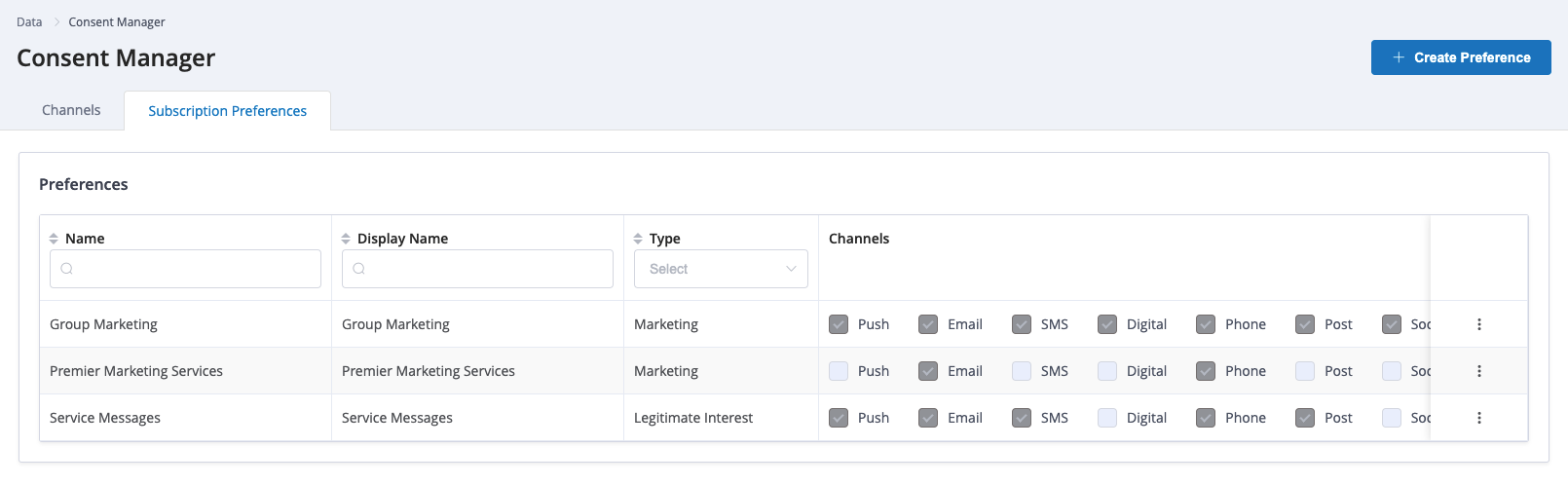
The example above shows three categories across a number of channels (Group Marketing, Premier Marketing Services and Service Messages).
Converted preference from subscription preference centre to Consent Manager
If you were using the existing Subscription Preferences feature, when the Consent Manager area rolls out your preferences will have been converted to the new format. This will not affect the use of your existing preferences, but the following rules will be applied:
- Any preferences with the exact same Name and Display name will be merged together as a single preference, with the different channels they were set up for checked for the new single preference. For example, if the existing set-up had three preferences all named casino_news with the Display Name blank for all of them, and one was selected for Email, one for SMS and one for Push, then after the update they would be merged into a single preference row as pictured in the screenshot above. This scenario applies if the Name and the Display name exactly match.
- Any preferences with the exact same Name but a different Display name will be merged as a single preference, but a message will show next to the preference (Update required) to allow users to provide a new Display Name.
Stored Display Names
If display names cannot be merged due to not matching, as described above, then the original display names will remain untouched in the database until the preference is edited and saved from the Subscription Preferences tab.
How to manage preferences for users
Email and SMS
From email and SMS campaigns
It is possible to add a link to the preference center to allow users to subscribe and unsubscribe to the different preferences from it. To be able to do so, navigate to Channels > Email Settings and Channels > SMS Settings and enable the Allow user to manage subscription preferences toggle.
After you do so and once you have created the different preferences, the Xtremepush email and SMS preference center will list all of these, which will allow users to opt-in or opt-out from them.
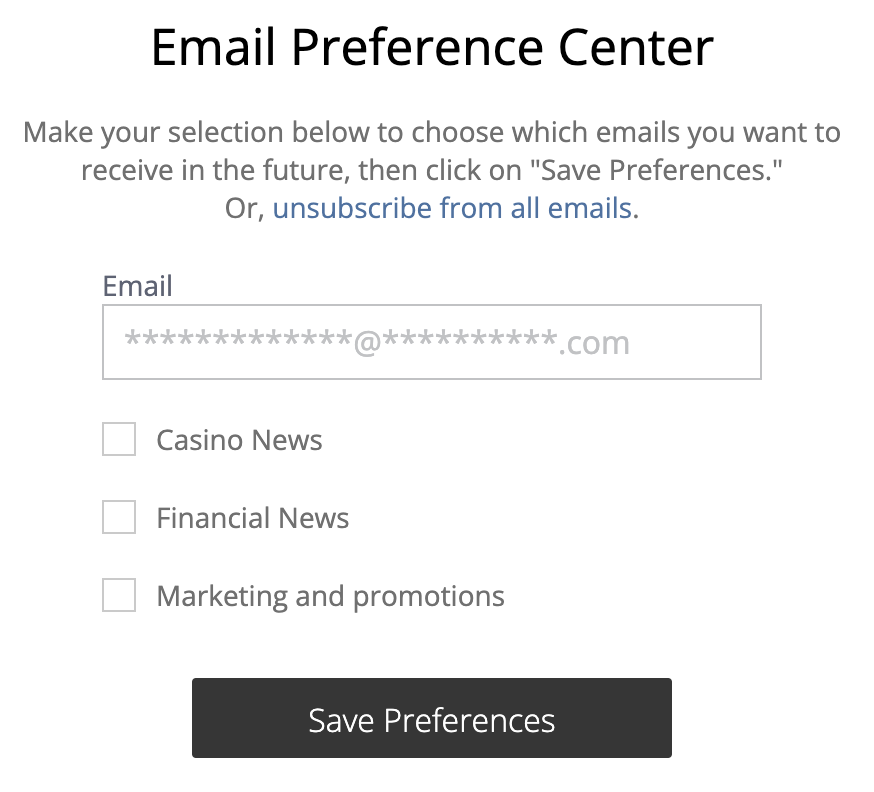
Email preference center showing different preferences to which users can subscribe to.
In email campaigns, users can access the email preference center if you include the following code as a hyperlink to the text:
{{system.url.profile}}
In SMS campaigns, you will need to include the unsubscribe link in your SMS message. That link will allow users to modify their preferences for specific categories.
Via import
It is now possible to update preferences via file import and via API.
It is only possible to set preferences in this way for profile-based channels (email, SMS or profile-based custom channels).
File import
To update preferences via file import, you'll need to include a column per preference and channel in your CSV and set each profile's value to true or false (1
or 0
). When importing Profiles from a CSV you can then map those columns in the file to preferences that exist in the project. The fields can be seen at the bottom of the Map to input column via: Data > Automations > Import (under Preferences).
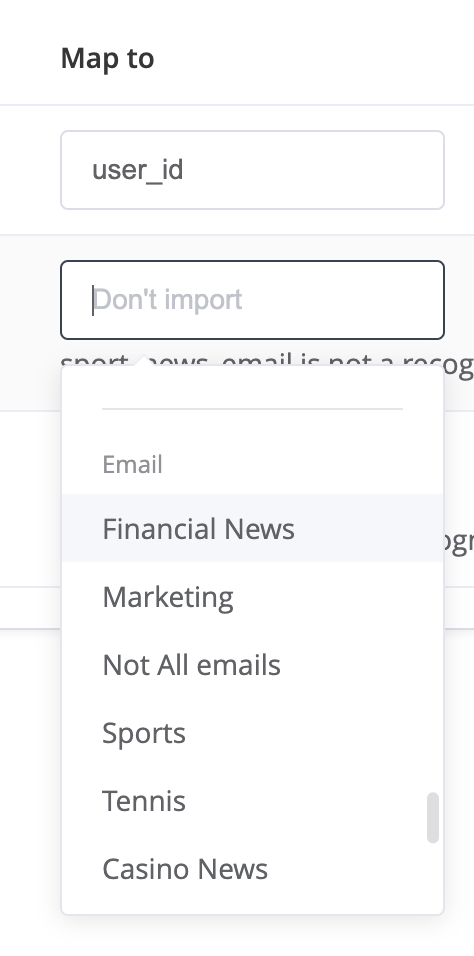
Mapping of a preference (Financial News) for the email channel.
API
The user profile import external API method can be used to set and update preferences, providing the Name of the specific preferences that need to be updated.
- Consent preferences are specified as a JSON object within the rows array.
- Each communication channel (e.g., SMS, email, custom_channel) is a key with nested preferences.
- The exact preference names must be obtained from the project these imports/updates are intended to be sent to.
- Use integers for consent values (e.g.,
0
for opt-out,1
for opt-in).
curl --request POST \
--url https://external-api.xtremepush.com/api/external/import/profile \
--header 'content-type: application/json' \
--data '{
"apptoken": "YOUR_APPTOKEN",
"columns": [
"user_id",
"consent_preferences"
],
"rows": [
"myuserid",
{
"email": {
"sport_news": 1,
"casino_news": 1
},
"sms": {
"sport_news": 0,
"casino_news": 1
}
}
]
}
Push channels (Android, iOS and web)
You can implement your own preference interface in your app or website and use our SDK methods to update the user profile's subscription when they select the different categories.
Device subscription
In order for devices to receive any push campaigns the standard push subscription must be enabled (which it is by default). Review our dedicated guide for more details.
JSONObject prefs = new JSONObject();
prefs.put("news", 1);
prefs.put("marketing", 0);
mPushConnector.updatePushSubscriptionPreferences(this, prefs, this);
let preferences = [
"news": true,
"marketing": false
]
XPush.updatePushSubcriptionPreferences(with: preferences) { (json: [String: Any], error: Error?) in
// handle response
}
[XPush updatePushSubscriptionPreferences: @{
@"news": @YES,
@"marketing": @NO
}
withCompletionHandler: ...];
xtremepush('user', 'update', {
'push_subscription_preferences': {
'news': 1,
'marketing': 0
}
},
function(data) {
console.log('success: ' + JSON.stringify(data))
},
function(data) {
console.log('error: ' + JSON.stringify(data))
}
);
From on-site campaigns
It is also possible to subscribe users to specific preferences from on-site campaigns, when they click on a specific button.
For example, you could create a campaign to encourage users to subscribe to your movies' newsletter.
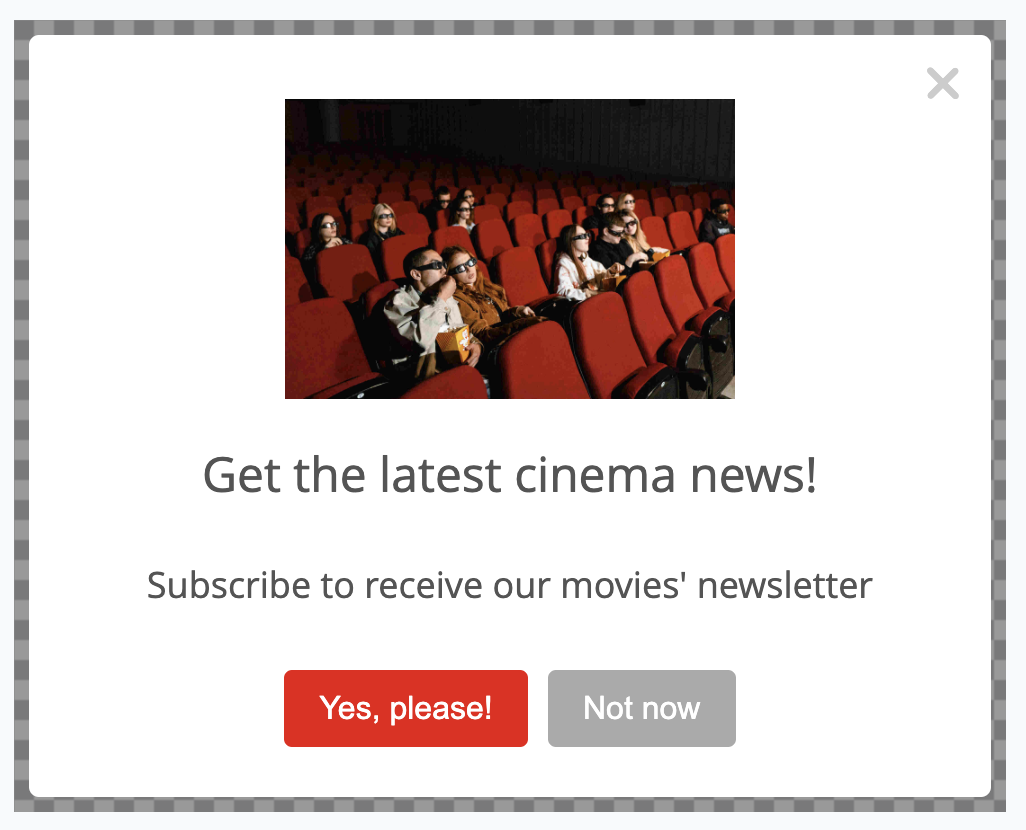
On-site campaign to get users subscribed to a 'movies' email newsletter.
To do so, navigate to Campaigns > Create a campaign > Single-stage > In-App/On-site message > select a pop-up style campaign. Fill in the required details from the Setup, Event, Segment and Schedule tabs. From the Content tab, include two buttons. On your subscription button, set the Subscribe field to the category that you created previously from the Consent Manager page.
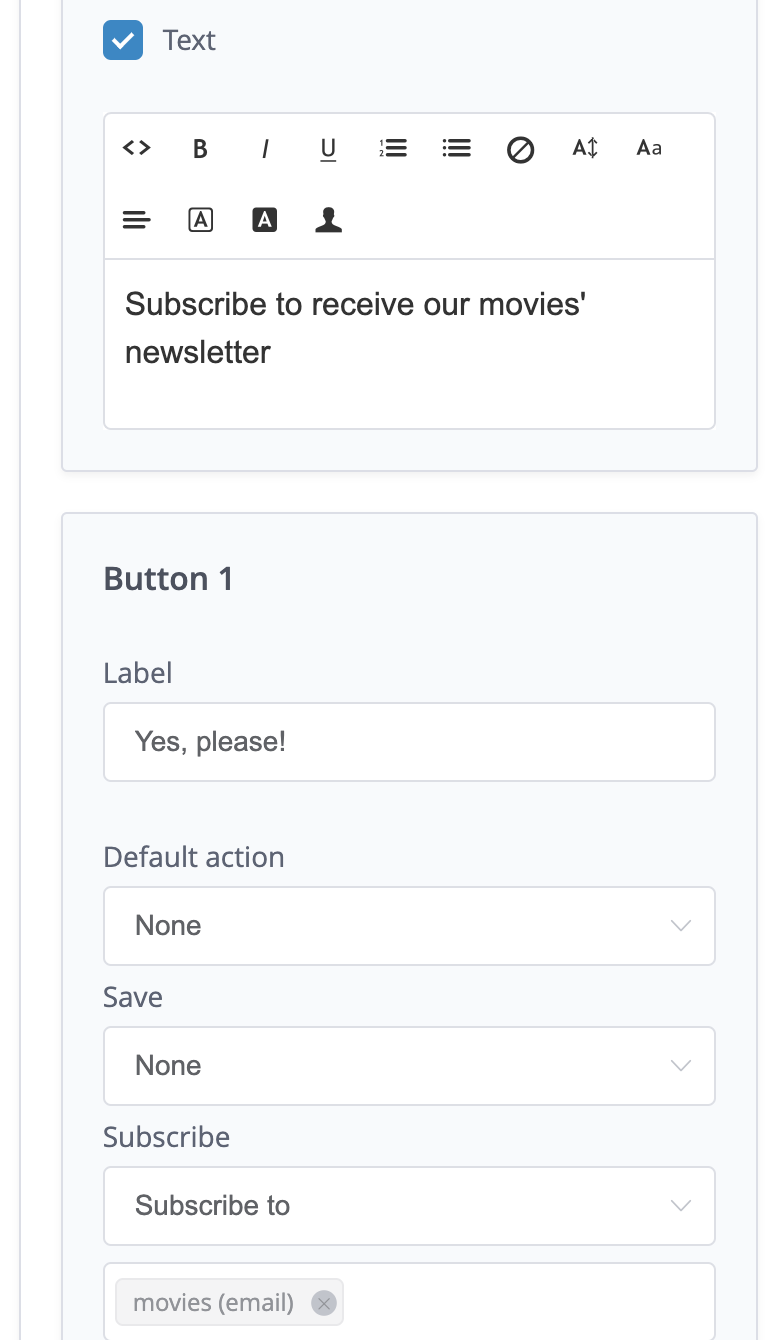
How to set up the campaign on the Xtremepush platform.
When users click on that button, they will automatically get subscribed to this category and channel.
From in-app campaigns
In a similar way as described above, users can opt in for push categories from in-app campaigns. This requires using the deeplink functionality.
To create the in-app campaign, navigate to Campaigns > Single-stage > Create a campaign > In-App/On-site message > select a full-screen or modal campaign type. Include two buttons, and on the desired button, from Action select the Go to deeplink option.
You can register a handler for this on Android and iOS to allow you to take action on the client side based on deeplinks. The deeplink payloads must have enough information to allow your app to make a decision on setting preferences. The deeplink format could be subpref://update?news=1
or subpref://update?news=1&marketing=1
.
See example below:
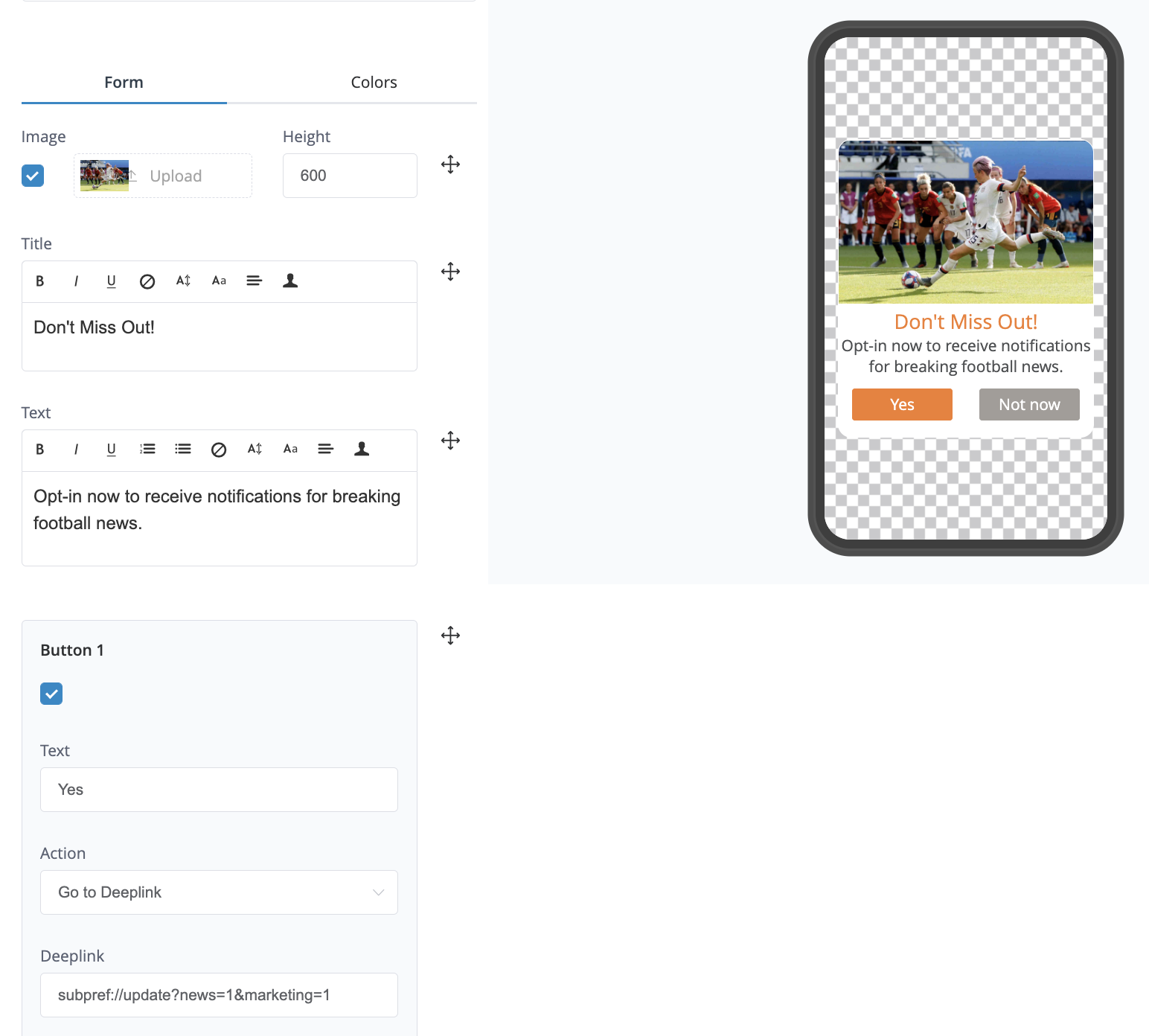
To review how to configure the handlers on Android and iOS, review the following sections of our Deeplink dedicated guides:
Additionally, here is an example based on parsing the deeplink with the format shown before; that would be a flexible way of setting the subscription preferences from in-app messages.
@Override
public void deeplinkReceived(String link, WeakReference<Context> uiReference) {
try {
//Turn string param into URI and break into relevant components
Uri uri = Uri.parse(link);
String host = uri.getHost();
String protocol = uri.getScheme();
Set<String> preferences = uri.getQueryParameterNames();
//Verify the protocol and host
if (protocol.equalsIgnoreCase("pushsubpref") && host.equalsIgnoreCase("update")) {
//create JSON objects.
//jo is the param we will be passing to the updateUser function
//myPushPreferences is some nested JSON in jo that represents the push params to update
JSONObject jo = new JSONObject();
JSONObject myPushPreferences = new JSONObject();
//loop through URI params then add each key-value pair to our 'myPushPreferences' JSON
for (String key : preferences) {
myPushPreferences.put(key, uri.getQueryParameter(key));
}
jo.put("push_subscription_preferences", myPushPreferences);
// if necessary also set the subscription to 1
jo.put("push_subscription", 1);
//call updateUser function.
//Params are a Context instance, the JSON object we described above, an XPCallbackHandler instance
mPushConnector.updateUser(this, jo, new XPCallbackHandler() {
@Override
public void onSuccess(JSONObject response) {
Log.d("TAG", "Here in on success. response : "+response.toString());
}
@Override
public void onFailure(JSONObject response) {
Log.d("TAG", "Here in on failure. response : "+response.toString());
}
});
}
} catch (Exception e){
}
}
XPush.registerDeeplinkHandler { x in
guard x.hasPrefix("pushsubpref"), let query = URLComponents(string: x)?.queryItems else { return }
var params: [String: String] = [:]
for q in query {
params[q.name] = q.value
}
XPush.updateUser(with: params) { jsonResponse, error in
<#code#>
}
}
[XPush registerDeeplinkHandler:^(NSString *x) {
if !([x hasPrefix:@"pushsubpref"]) {
return;
}
NSMutableDictionary* params = @[].mutableCopy;
for (NSURLQueryItem* q in [NSURLComponents componentsWithString:x].queryItems) {
params[q.name] = q.value;
}
id prefs = @{ @"push_subscription_preferences": params,
@"push_subscription": @(1)
};
[XPush updateUserWith:prefs completionHandler:^(NSDictionary * _Nullable jsonResponse,
NSError * _Nullable error) {
<#code#>
}];
}];
Review users' subscribed categories
Profile preferences
From Data > User profiles it is possible to review users' individual preferences and subscription to profile-based channels by navigating to the Channels & Preferences tab. The subscriptions to each preference and channel shows up under the Preferences section.
Subscription to the email/SMS channel is separate to subscription to specific preferences. Even if a user is not subscribed to any specific category, if the user is subscribed to email/SMS, the green tick will show against the channel. This status can be altered manually by clicking the Options menu > Unsubscribe.

Click each channel tab to see the preferences the user profile is subscribed to within that specific channel. To manage the individual subscriptions to each category click on the Options menu on each of the line items.
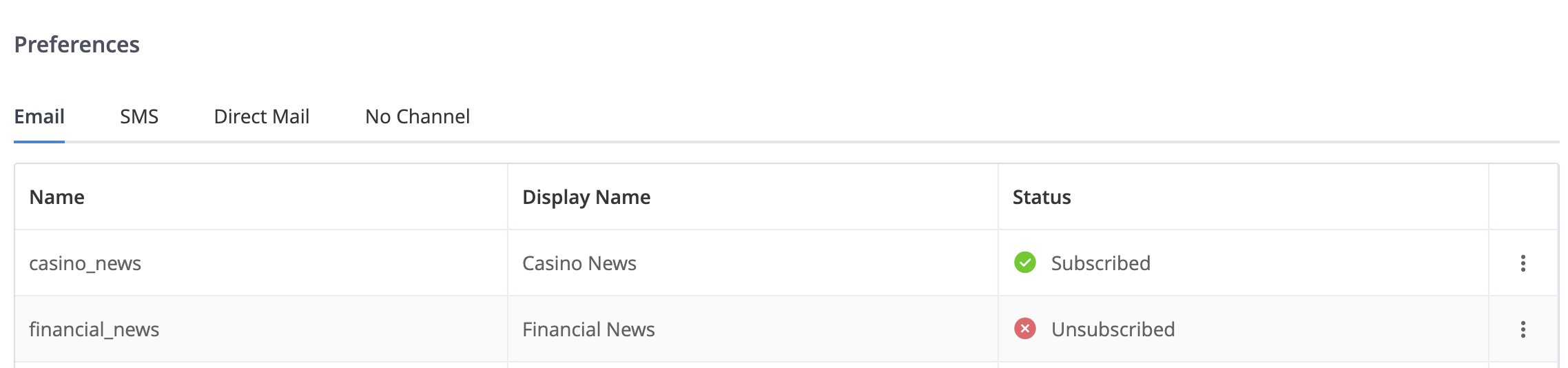
For the email channel, this user is unsubscribed from 'Financial News' and subscribed to 'Casino News'.
Note the section for No Channel where non-channel related preferences are available to check.
Device preferences
These can be reviewed and managed via the Push Subscriptions section. Expanding each device row shows the related preference for that specific device. These can be managed by selecting the Options menu and updating the subscription from there.
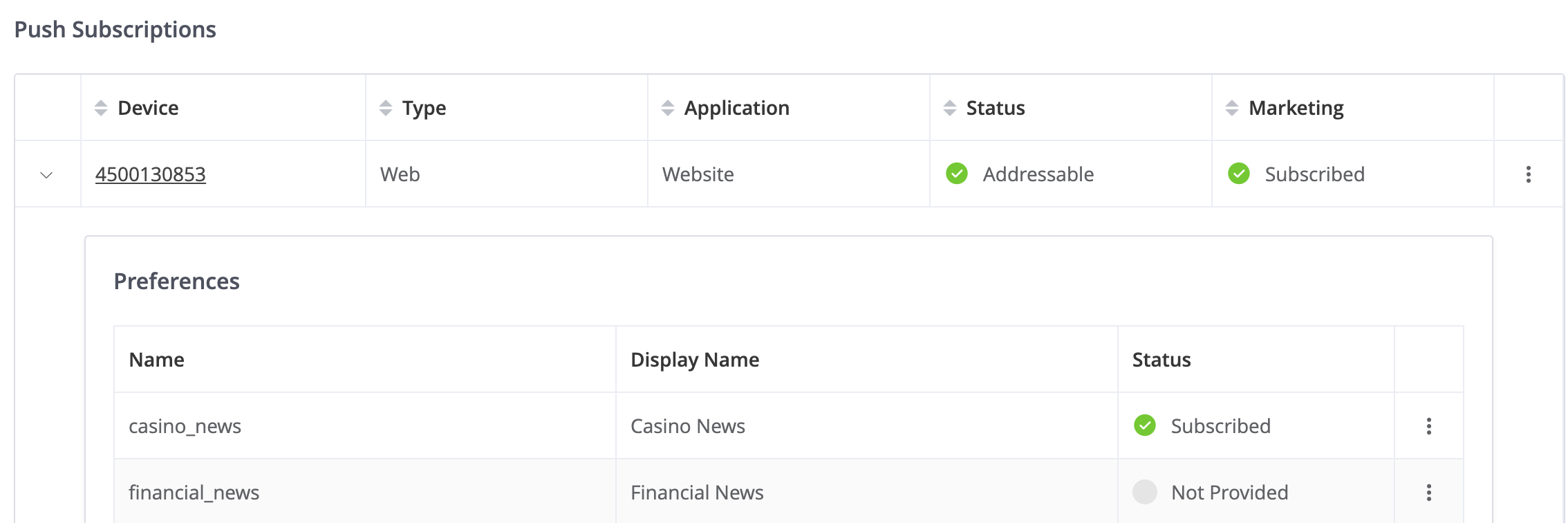
This web device is subscribed to 'Casino news'. They haven't stated whether they would like to be subscribed to 'Financial News'.
Segment based on subscription preferences
It is possible to segment based on users' subscription status to the different categories. To do so, either from the segmentation on a campaign level (from the Segment tab) or directly from the segmentation engine (Data > Audiences > Segments > Create a segment), select the condition Engagement > Subscription Prefs. Select the desired category. It will be possible to segment based on who is subscribed or not subscribed to that specific category.

The example above shows a segmentation by users subscribed to the category 'Nature' for push channels.
You can also include consent preferences when exporting the segment.
Navigate to Audiences → Segments.Select a segment, click on the three dots menu, and choose Export Users → Users.
In the Customize Export popup, you’ll find an Export Preferences section. Use this to add the consent preferences relevant to your needs.
Link preferences to campaigns
Linking consent preferences to campaigns is essential for ensuring regulatory compliance and improving the relevance of your communications. This feature allows marketers to align campaigns with user-provided consent preferences, ensuring messages are only sent to users who have opted in for specific channels (e.g., Email, SMS, Push).
Single-Stage Campaigns
When creating a new campaign on the Setup step, you will have the option to toggle on the Preference Consent feature, as demonstrated below. Once the feature is toggled on, you can select an existing preference from the Preferences Dropdown or choose to Ignore Subscription.

Consent Preferences toggle in the campaign Setup tab
Multi-Stage Campaigns
For each message in the campaign journey, you must select the subscription preferences independently. This ensures that each stage aligns with the correct preference.
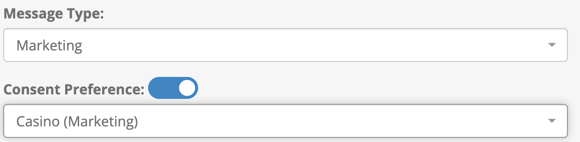
Consent Preferences toggle in the Multi-Stage Campaign message setup.
Validation when Consent Preference is enabled
The platform automatically validates consent preferences at the point of send:
- Consent preference enabled: then the specific preference selected will be used to determine whether the User should be sent the campaign and for the relevant channel. Users without explicit consent for the selected preference and channel will be excluded from the campaign. Global subscription is ignored.
- Consent preference disabled: then the User's Global channel preference will be checked.
Be aware that after May 1st 2025 for sports betting and igaming clients this may not be a sufficient enough check to stay within the UKCG guidelines. You should check the details to make sure you are remaining compliant. The responsibility to do this is on you, not the Xtremepush platform. - For ignore subscriptions, the message will be sent to all users.
Unsubscribe Link Behavior
Email Campaigns
- Consent management is enabled in your project and consent preference is configured in the campaign
Clicking the Unsubscribe link allows the user to unsubscribe only from the specific consent preference associated with the campaign. Clicking the Unsubscribe from all link unsubscribes the user from the global email subscription. All email communications are disabled, regardless of preferences.
- Consent management is enabled in your project but consent preference is not configured in the campaign
Clicking the Unsubscribe from all link unsubscribes the user from the global email subscription. All email communications are disabled, regardless of preferences.
- Consent management is disabled
Clicking the Unsubscribe link unsubscribes the user from the global email subscription. All email communications are disabled, regardless of preferences.
SMS Campaigns
- Consent Preferences Enabled: Clicking the unsubscribe link directs users to the Preference Center, where they can manage their SMS preferences.
- Consent Preferences Disabled: Same as above
- SMS unsubscribe requires two actions:
- Users select preferences to unsubscribe from in the Preference Center.
- They confirm by clicking "Save."
Push Notifications
Push notifications typically do not include a direct unsubscribe link. Instead:
- Campaigns can redirect users to the Preference Center or app settings, allowing them to manage preferences.
- Users can unsubscribe from preferences tied to the campaign while retaining other notifications.
Additional Information
- Consent preferences cannot be edited after the campaign has been launched.
- Each campaign is linked to one consent preference to ensure clarity and compliance, you cannot add multiple preferences in one single-stage campaign.
- If users unsubscribe during an active campaign, the audience is dynamically updated to exclude unsubscribed users during the campaign lifecycle.
- If the recipient clicks through to the preference centre and clicks opt out from all then they will be opted out/unsubscribed from all preference and the Global subscription.
- If the user is opted out from all preferences and unsubscribed from the Global subscription but they then use the preference centre to opt back in to any preference they will also be resubscribed to the Global subscription.
Updated about 1 month ago